public class StringReverse {
public static void main(String[] args) {
String str="selenium",reverse="";
for(int i=str.length()-1;i>=0;i--) {
reverse=reverse+str.charAt(i);
}
System.out.println(reverse);
}
}
Output: muineles
...
Monday, December 31, 2018
Difference between Hashmap and Hashtable?
HashMap is non-synchronized. Hashtable is synchronized.
HashMap allows multiple threads where as hashtable doesn't
HashMap is not thread-safe where as hashtable is.
HashMap allows one null key and any number of null values. Hashtable doesn’t allow null keys and null values.
Note: We can make the HashMap as synchronized by calling this code
Map m = Collections.synchronizedMap(hashMap) [or]...
Difference between ArrayList and LinkedList?
Similarities: Both implements List interface(linkedlist also implements deque interface), maintains insertion order and can contain duplicate elements. Both are non synchronized classes can be made synchronized explicitly by using Collections.synchronizedList method .
Differences:
ArrayList internally uses a dynamic array to store the elements. LinkedList internally uses a doubly...
Difference between Arraylist and HashMap?
ArrayList implements List Interface while HashMap is an implementation of Map interface.
Memory consumption is high in HashMap compared to the ArrayList.
ArrayList maintains the insertion order while HashMap doesn't maintain any order. ArrayList stores the elements only as value and maintain internally the indexing for every element. While HashMap stores elements with key and value pair, i.e. two objects....
Saturday, December 29, 2018

System.out.println(). Simply SOP
System: is a final class in java.lang package. This class has methods to provide access to Standard Input, Standard Output and Standard Error Output streams. Can not be instantiated.
out: is final static variable of type PrintStream declared in the System class.
println(): is a overloaded method in PrintStream class. It accepts...
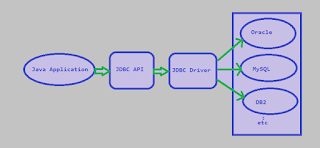
JDBC: is an acronym for 'Java Data Base Connectivity'. It is used for accessing the databases from Java Applications. JDBC API allows to do CRUD operations on Database.
Steps to connectivity between Java program and Database:
Loading the Driver
Create the Connections
Create a Statement
Execute the Query
Close the Connections.
Loading the Driver:
We can register...
Saturday, December 22, 2018
Thursday, December 20, 2018
Marker Interface:
A marker interface is an empty interface with no fields or methods. These are used to indicate some information to compilers or JVMs
Ex: Serializable, Cloneable, EventListener and Remote interfaces
A Class implements them to claim the membership in a particular set.
The String class and all the wrapper classes implement the java.io.Serializable interface by defau...
Tuesday, December 4, 2018

I have attended some interviews during my career. I am sharing with you questions i was asked in the interviews. Hope these questions are helpful to the readers. A Java Program to retrieve the data from an excel file? Difference between Arraylist and HashMap? Difference between ArrayList and LinkedList? Difference...
Subscribe to:
Posts (Atom)